React, also known as ReactJS, was born as a Facebook javascript library (not a framework) for building user interfaces. It was born to facilitate the creation of interactive and reusable UI components. However it performs on both client and server side, providing interoperability. React was specifically built for handling complex DOM interactions/updates. It allows agile development, neat, with a maintainable architecture focused on the performance.
Why, how, when ? History
React started as a JavaScript port of XHP, a version of PHP released by Facebook. Its main concern was to minimize Cross Site Scripting (XSS) attacks (a malicious user enters content that is intended to inflict harm on the viewer of that content). However the problem with XHP was that dynamic applications require many roundtrips to the server, that is why an engineer at Facebook took XHP into the browser using Javascript, and created ReactJS.
React is used not only at Facebook. Instagram was entirely coded using React, and many other sites and apps are now adopting React.
What does React solve ?
React helps to improve the performance of the applications. DOM operations reduces the performance of the applications making them to render slowly when there are much operations. React will render wherever is necessary, without affecting or refreshing the other areas. It avoids rendering unnecessary pages and nodes by utilizing Virtual DOM.
What is the advantage of Virtual DOM ?
Virtual DOM works by rendering subtrees of nodes based on state changes. It keeps the components updated while also avoiding the manipulation of nodes when it’s not necessary.
Virtual DOM proceeds with a two steps work part of the object is changed. Instead of rebuilding the whole object, it will run an algorithm that will compare a mirrored object with the one that changed and find the differences. Then it will only render the nodes where those differences are. This way, React improves considerably the performance.
How is a React application organized ?
It uses component that represent different UI parts. Each component has the necessary markup and script to be completely functional. By coding modular components, we can reuse the code in the same app or a different one.
Each component has a set of properties and a state. Components can be nested.
Working with Flux
Facebook also designed an architecture concept, Flux, to work with React. This architecture is for creating data layers in JavaScript applications, and focuses on creating explicit and understandable update paths for the application's data, making trace changes during development simpler at the same time that bugs are easier to track down and fix.
Facebook came out with Flux as an attempt to solve the problems caused by the MVC pattern in their codebase. They struggled with actions triggering cascading updates that led to unpredictable results and code that was hard to debug, due to the tightly coupled code.
Flux breaks down into four major parts: actions, the dispatcher, stores, and views
- Actions: describe an action that takes place in the application.
- Dispatcher: is a singleton registry of callbacks. It acts passing the actions to all the stores that are subscribed to it.
- Stores: manage the state and logic needed to update specific parts of the application.
- Views: are React components.
In Flux, all data flows in a single direction: Actions are passed to the Dispatcher using convenience classes called Action Creators. The dispatcher sends the actions to all the stores that are subscribed to it. And finally, if the stores care about a particular action that was received (or more), they update their state and signal the views so they can re-render.
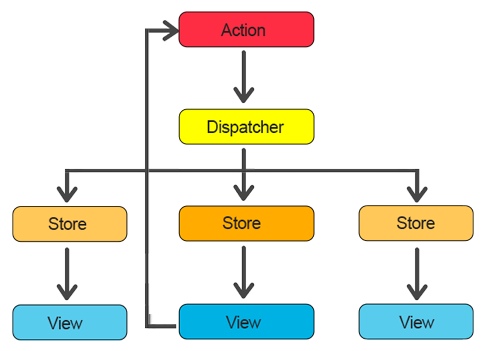
In Flux, the Dispatcher is a singleton that directs the flow of data and ensures that updates do not cascade. That way, as the application grows, the dispatcher manages dependencies between stores by invoking the registered callbacks in a specific order. Flux works well with ReactJS because the single directional data flow makes it easy to understand and modify an application as it becomes more complicated. Two-way data bindings lead to cascading updates, where changing one data model led to another data model updating, making it very difficult to predict what would change as the result of a single user interaction.
When a user interacts with a ReactJS view, the view sends an action through the dispatcher, which notifies the various stores that hold the application’s data and business logic. When the stores change state, they notify the views that something has updated.
Flux is more of a pattern than a formal framework, but the same term also refers to a library that represents the official implementation.
Flexibility
React can be used with other frameworks/libraries, such as JQuery, Backbone, or Angular.
If we are starting a project, we can build it on the Flux architecture. If we already have an ongoing project using an MVC Framework, as AngularJS, we can keep AngularJS as a controller, and ReactJS to take care of the views. ReactJS has a superior performance when manipulating DOM elements, which has a big impact when dealing with constant changes in our visualization.
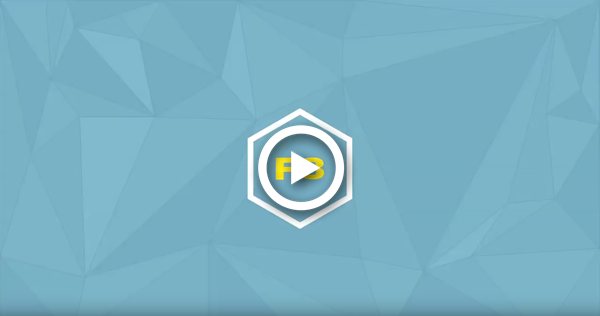
Rethinking Web App Development at Facebook
Is React good for your application ?
The main questions are:
- Is the user interaction going to affect many other areas ?
- Is my data going to change over the time at a frequent high rate ?
- Is my application going to keep growing big ?